Maven
- 자바용 프로젝트 관리 도구
- 프로젝트의 전체적인 라이프 사이클을 관리하는 도구
- 프로젝트가 커지고 프로젝트 수가 증가하면서 프로젝트간의 의존관계가 발생하고, 오픈 소스 프레임워크의 활용이 증가됨에 따라 프로젝트가 관리해야 하는 외부 라이브러리 수가 증가되었다.
- 이 같은 이유로 개발자들이 정작 신경 써야 할 핵심 비즈니스 로직보다 복잡한 프로젝트 구조와 라이브러리 관리에 많은 시간을 투자하게 되었다. 이러한 문제를 해결하기 위해 Maven의 필요성이 대두되었다.
- Maven을 사용하면 의존관계 라이브러리를 편리하게 관리할 수 있다.
- Maven은 필요한 라이브러리를 특정 문서(pom.xml)에 정의해 놓으면 내가 사용할 라이브러리뿐만 아니라 해당 라이브러리가 작동하는 데 필요한 다른 라이브러리들까지 관리하여 네트워크를 통해서 자동으로 다운로드 받아 준다.
Maven 설정 파일
- setting.xml
- 메이븐 설치시 기본으로 제공되는 XML (MAVEN_HOME/conf/ 에 위치함)
- 메이븐 툴과 관련된 설정 파일
- pom.xml
- 프로젝트에 필요한 라이브러리를 정의하는 공간
- POM: Project Object Model => 프로젝트의 Object Model의 정보를 담고 있는 파일
- 해당 라이브러리가 작동하는 데 필요한 다른 라이브러리 자동 설치
[pom.xml 예시]
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
|
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.12</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>SpringWeb</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>SpringWeb</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>com.oracle.database.jdbc</groupId>
<artifactId>ojdbc11</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter-test</artifactId>
<version>3.0.3</version>
<scope>test</scope>
</dependency>
<!-- Add MyModule -->
<!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-tomcat -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<scope>provided</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.tomcat.embed/tomcat-embed-jasper -->
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
<!-- https://mvnrepository.com/artifact/javax.servlet/jstl -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.2</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-fileupload/commons-fileupload -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.3</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-io/commons-io -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.4</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<image>
<builder>paketobuildpacks/builder-jammy-base:latest</builder>
</image>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
</project>
|
cs |
STS
- STS(Spring Tool Suite): 이클립스 기반으로 만들어진 스프링 애플리케이션 개발용 도구
- STS는 기본적으로 Maven을 내장하고 있다.
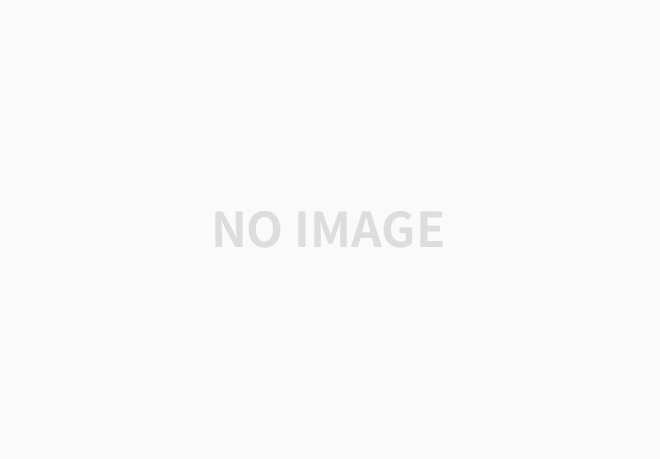
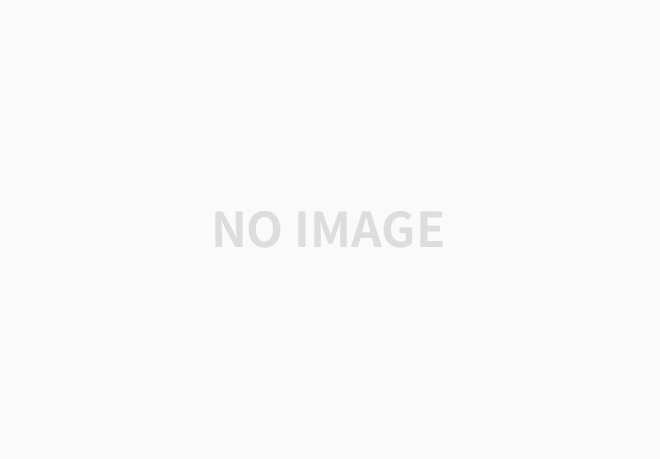
구성요소 | 설명 |
src/main/java | 작성되는 코드의 경로로, 자바 소스 파일 위치 |
src/main/resources | 실행할 때 참고하는 기본 경로 (주로 설정 파일들을 넣음) ex. 프로퍼티 파일, XML 파일 |
src/test/java | JUnit 등 테스트 코드를 넣는 경로 |
src/main/webapp | WEB_INF 등 웹 애플리케이션 리소스 위치 |
pom.xml | Maven이 사용하는 pom.xml 프로젝트 정보가 표시되며 스프링에서 사용되는 여러가지 라이브러리를 설정해 다운로드할 수 있음 |